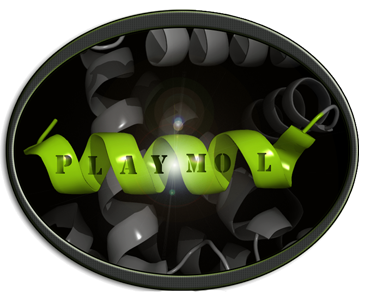
Here is a collection of code snippts and examples that I find useful and efficient (some require additional packages).
Content:
Here is an example where I create the temperature folders 275, 280, 285, 290, 295, 300, 305 and 310 and 20 subfolders for each temperature:
for T in $(seq 275 5 310);do for sub in {1..20};do mkdir $T/$sub done doneAnd that is all. Now it is easy to use the same idea to copy your MD jobs to each folder, submit them, and later extract results from them.
echo "2.2 + 3.1"
This will however just return "2.2 + 3.1" and not "5.3" as we want. It is possible to do math within the BASH environment, but then we need to use bc:echo "2.2 + 3.1" | bc
which returns "5.2" as we wanted it to. However, if you type:echo "sqrt(3)" | bc
"1" is returned. This is because we need to specify how many decimals we want. Since the input is 3, this is taken as an integer, and that is also what is returned, even though we want a float. Use scale to specify how many decimals you want:echo "scale=3; sqrt(3)" | bc
This will return "1.732".for i in *.png;do echo "$i"; convert "$i" -resize 100x "$i";done
100x resizes the width to 100 px and the height to the corresponding aspect. To resize based on the height, you just type x100 to obtain a heigth of 100 px:for i in *.png;do echo "$i"; convert "$i" -resize x100 "$i";done
Here is one example to combine two images side by side:
convert file1.png file2.png +append -quality 75 output.png
Here is one example to combine images on top of each other using layers:
convert -page 800x600 background.png -page +650+30 image1.png -layers flatten output.png
Here image1.png is placed on top of the image called background.png. The "+650+30" is the placement of image1.png relative to the first image (background.png). With '-page' you can place as many images as you like on top of each other.